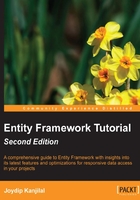
Creating the EDM
Now that the Security
database is ready, we will explore how we can create an EDM on top of the Security
database.
Note
Note that, before Entity Framework 7, there were two storage models—the EDMX file format based on XML schema or code. With Entity Framework 7, the EDMX file format will be dropped—we will have only the code-based format. Interestingly, this approach is also termed the "code-first only" approach.
You can create the Entity Data Model in one of two ways:
- Use the ADO.NET Entity Data Model Designer
- Use the command-line Entity Data Model Designer called
EdmGen.exe
The first approach is preferred to the second. However, as we move through the chapters of the book, we will explore how we can follow the code-first approach to implement the model for our application that uses Entity Framework.
We will first take a look at how we can design an EDM using the ADO.NET Entity Data Model Designer.
Creating the Entity Data Model using the ADO.NET Entity Data Model Designer
To create an EDM using the ADO.NET Entity Data Model Designer, follow these simple steps:
- Open Visual Studio.NET 2013 IDE, create a solution for a new web application project as follows, and save it with a name.
- Switch to the Solution Explorer, and navigate to Add | New Item... to create a new Entity Data Model using Entity Data Model Wizard.
- Next, select ADO.NET Entity Data Model from the list of templates displayed, as shown in the following screenshot:
Creating a new ADO.NET Entity Data Model
- Name the Entity Data Model
SecurityDB
, and click on Add. - Select Generate from database from Entity Data Model Wizard, as shown in the following screenshot:
Generating the Entity Data Model from the database
If you select the Empty model template and click on Next, the following screen appears:
Empty Entity Data Model Wizard
As you can see from the previous screenshot, you can use this template to create the EDM yourself.
We will not use this template in our discussion here, so let's get to the next step.
- Click on Next in the Entity Data Model Wizard window shown earlier.
- The modal dialog box will now appear and prompt you to choose your connection.
- Click on New Connection. Now you will need to specify the connection properties and parameters for the database to connect to. In our example, the database is
Security
.Note
We will use a dot to specify the database server name. This implies that we will be using the database server of the localhost, which is the current system in use. You can also specify the server name here if your database resides on a different system. If your database resides on a different server, you need to specify the server name here.
- After you specify the necessary user name, password, and server name, you can test your connection using the Test Connection button. When you do so, the message Test connection succeeded gets displayed in the message box, as shown in the following screenshot:
Testing the database connection
Note
Note that the entity connection string is generated automatically. This connection string will be saved in the
ConnectionStrings
section of your application'sweb.config
file. This is what it will look like:<connectionStrings> <add name="SecurityDBEntities" connectionString="metadata=res://*/SecurityDB.csdl|res:/ /*/SecurityDB.ssdl|res://*/SecurityDB.msl;provider=System.Data.SqlClient;provider connection string="data source=.;initial catalog=SecurityDB;user id=sa;password=sa1@3;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" /> </connectionStrings>
- Now, click on Next and specify the database objects you would like to have in your model from the Choose Your Database Objects and Settings window that is shown next:
Choosing the database objects to be used in the model
We will select all the tables of the
Security
database now. Refer to the following screenshot:Selecting the Database Objects
- Lastly, click on Finish to generate the EDM for the
Security
database.
Your EDM has been generated and saved in a file named SecurityDB.edmx
. We are done creating our first EDM using the ADO.NET Entity Data Model Designer tool.
When you open SecurityDB.edmx
that we just created in the designer view, it will appear as shown in the following image:

The SecurityDB Entity Data Model
In the next section, we will learn to create an EDM using the EdmGen.exe
command-line tool.
Creating Entity Data Model using the EdmGen tool
We will now take a look at how to create a data model using the EDM generation tool called EdmGen.
The EdmGen.exe
command-line tool can be used to do one or more of the following:
- Generate the
.cdsl
,.msl
, and.ssdl
files as part of the EDM - Generate object classes from a
.csdl
file - Validate an EDM
The EdmGen.exe
command-line tool generates the EDM as a set of three files: .csdl
, .msl
, and .ssdl
. If you have used the ADO.NET Entity Data Model Designer to generate your EDM, the .edmx
file generated will contain the CSDL, MSL, and the SSDL sections. You will have a single .edmx
file that bundles all of these sections into it. On the other hand, if you use the EdmGen.exe
tool to generate the EDM, you will find three separate files with .csdl
, .msl
, or .ssdl
extensions.
Here is a list of the major options of the EdmGen.exe
command-line tool:

Note that you need to pass the connection string, and specify the mode and the project name of the artifact files (the .csdl
, .msl
, and .ssdl
files) to be created. To create the EDM for our database, open a Visual Studio command window and type in the following:
edmgen /mode:fullgeneration /c:"Data Source=.;Initial Catalog=SecurityDB;User ID=sa;Password=sa1@3;" /p:SecurityDB
This will create a full Entity Data Model for our database. The output is shown in the following screenshot:

Generating the Entity Data Model from the command line
You can also validate the SecurityDB
model that was just created, using the ValidateArtifacts
option of the EdmGen command-line tool, as follows:
EdmGen /mode:ValidateArtifacts /inssdl:SecurityDB.ssdl /inmsl:SecurityDB.msl /incsdl:SecurityDB.csdl
When you execute the preceding command, the output will be similar to what is shown in the previous screenshot.
As you can see in the previous screenshot, there are no warnings or errors displayed. So, our EDM is perfect.
The section that follows discusses the DataSource controls included in ASP.NET and also the new EntityDataSource control, which was first introduced as part of the Visual Studio.NET 2008 SP1 release. Note that the EntityDataSource control is included as part of Visual Studio 2010 and onward.