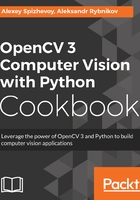
上QQ阅读APP看书,第一时间看更新
How to do it...
Use the following steps:
- Import all necessary modules:
import cv2
import numpy as np
import matplotlib.pyplot as plt
- Load the image as grayscale and display it:
grey = cv2.imread('../data/Lena.png', 0)
cv2.imshow('original grey', grey)
cv2.waitKey()
cv2.destroyAllWindows()
- Equalize the histogram of the grayscale image:
grey_eq = cv2.equalizeHist(grey)
- Compute the histogram for the equalized image and show it:
hist, bins = np.histogram(grey_eq, 256, [0, 255])
plt.fill_between(range(256), hist, 0)
plt.xlabel('pixel value')
plt.show()
- Show the equalized image:
cv2.imshow('equalized grey', grey_eq)
cv2.waitKey()
cv2.destroyAllWindows()
- Load the image as BGR and convert it to the HSV color space:
color = cv2.imread('../data/Lena.png')
hsv = cv2.cvtColor(color, cv2.COLOR_BGR2HSV)
- Equalize the V channel of the HSV image and convert it back to the RGB color space:
hsv[..., 2] = cv2.equalizeHist(hsv[..., 2])
color_eq = cv2.cvtColor(hsv, cv2.COLOR_HSV2BGR)
cv2.imshow('original color', color)
- Show the equalized full color image:
cv2.imshow('equalized color', color_eq)
cv2.waitKey()
cv2.destroyAllWindows()